Java Development: Some Development Particularities
In your Java implementation, you need to respect the particularities listed below. If you want to provide an existing Java library as an xUML library for the Designer, you need to rework your Java implementation regarding these points.
Java Version
For the provided setup to work, gradle has to be run with Java 11, but the jar files generated have to be Java 8 compatible. This means you need to apply the following settings to your development environment:
Java SDK needs to be set to a Java 11 SDK.
Language level should be set to version 8.
Java Code Particularities
Classes
The following must apply to all Java classes you want to expose to an xUML service:
The classes you want to expose to the user through the xUML library have to be annotated with an annotation @XumlLibraryClass
, to define the name under which the class should be exposed:
@XumlLibraryClass(name = "HelloWorld")
public class HelloWorldExample {
...
The DevKit contains a Java class HelloWorldExample. The annotation defines that this class should be exposed in the xUML library under the name of HelloWorld:
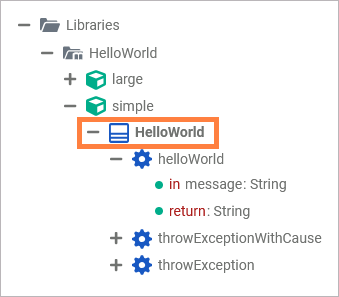
Important: The Java class and the xUML library class from the annotation must not have the same name.
These Java classes also need to follow the JavaBeans™ specification.
The class need to be declared public and have a public default constructor (zero-argument constructor, see the LargeObject class from the DevKit):
CODEpublic class LargeObject { private final String id; private final List<String> values; public LargeObject() { ...
The class must have private fields only with public getter/setter methods. In your xUML service implementation, you can access objects of such a class using the dot notation. The getter/setter methods are then called automatically.
The types of the class attributes must be able to map either to xUML base types, or have a default constructor themselves.
Methods
All methods from exposed classes have to be declared public and must be static only. Other, private or non-static methods are not possible.
Parameters
For all method parameter and return types, the following conditions need to be fulfilled:
Either, the type can be mapped to an xUML base type (see Type Mapping further below),
or, the type holds a public default constructor (zero-argument constructor). The class attributes must have corresponding getter and setter methods (see Classes above).
The names of the parameters are derived from the Javadoc:
/**
* Returns the provided message or a default message.
*
* @param message Provide input for the message to be returned. Defaults to "Hi from the Scheer PAS team!".
* @return Returns the message entered.
*/
public static String helloWorld(String message) {
...
Use the
@param
followed by the parameter name (e.g. message in the example above) to define the exposed parameter name.Name and order of parameters in the Javadoc must match the name and order of parameters in the Java implementation.
In the Designer, you can find the parameters accordingly:
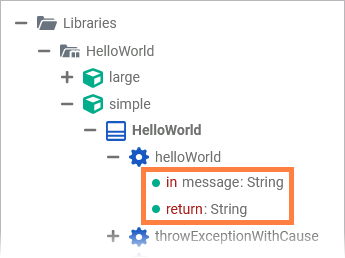
Parameter Troubleshooting
If name and/or order of the parameters do not match, or
if the Javadoc version is not Java 11 (see Java Development: Setting-up the Development Project),
the parameter names can not be generated correctly, and will be derived from parameter type (e.g. string, string1, …).
Type Mapping
The following table shows all possible mappings of Java data types to xUML base types.
Java Type | xUML Base Type |
---|---|
Arrays except byte[] and java.lang.Byte[] | Array |
boolean, java.lang.Boolean | Boolean |
byte, java.lang.Byte | Integer |
byte[], java.lang.Byte[] | Blob |
char, java.lang.Character | String |
double, java.lang.Double | Float |
float, java.lang.Float | Float |
int, java.lang.Integer | Integer |
java.io.InputStream and subclasses | Blob |
java.lang.String | String |
java.util.Collection and implementations | Array |
java.util.Date and subclasses | DateTime |
java.util.GregorianCalendar and subclasses | DateTime |
java.util.Map and implementations | Array of a specialized class with stereotype MapEntry, having two attributes of xUML base type |
long, java.lang.Long | Integer |
short, java.lang.Short | Integer |
The java.io.File class cannot be mapped, as it is not known if it is used as input or output.
Arrays of InputStream are not supported.
If a Java class does not apply to the requirements listed above, you can write your own Java wrapper class instead without modifying the original class. If the wrapper class applies to the import preconditions, you can import it instead.
Some Development Hints
Checked exceptions in the default constructor
The PAS Java wrapper cannot handle checked exceptions in the default constructor of a Java class. If you need this nevertheless, you need to wrap the checked exception with an unchecked one.Data manipulation on the Java object
If you want to manipulate data of a Java object, there is no need to transfer it to xUML and back (if not necessary). Leave the object management to Java and store the created objects in a thread-save concurrent hashmap. Then, simply transfer only an object id between the xUML model and the Java implementation (see also the LargeObject example from the template).Catching exceptions after adapter call
If you want to catch exceptions that occurred in a Java method in your Designer model, you need to throw these exceptions in your Java code using one of the following:CODEthrow new BridgeException("your error message", "your error code", "your error domain"); throw new BridgeException("your error message", "your error code"); throw new BridgeException("your error message");
In order to use
BridgeException
, you need to import class ch.e2e.bridge.server.BridgeException from addon.jar to your Java implementation (see also the simple.Operations example from the template).
xUML Objects and Java Objects
Please consider the following facts on xUML and Java classes, and their mapping when modelling xUML services:
Objects passed between Java and xUML worlds are mapped. There are no references to the original instances retained.
You cannot create a Java object in an xUML model.
Library Documentation
You can and should add documentation to your Java xUML library. The library documentation can be displayed from the asset repository, and it helps a user to decide whether and how they can use the library.
Documentation of a Java xUML library is twofold. You can provide a general description of your library, and you can add documentation to every exposed method.
General Description
In your Java template, you can find a file description.html. This file should contain a general library description. Replace the template content with your own content. You can use normal HTML to provide a comprehensive introduction to what your library does and how it is supposed to be used.
Find below the description of the HelloWorld example:
<!DOCTYPE html>
<html>
<body>
<div class="center_div">
<h1>Library Description</h1>
<p>Enter a description of your library here. This description will be visible in the Designer in the library documentation.</p>
</div>
</body>
</html>
In the Designer, you can find this piece of documentation on the library documentation page:

Documentation via Javadoc
All other documentation besides the general description is done via the Javadoc syntax.
/**
* Returns the provided message or a default message.
*
* @param message Provide input for the message to be returned. Defaults to "Hi from the Scheer PAS team!".
* @return Returns the message entered.
*/
public static String helloWorld(String message) {
...
Java Classes
Use Javadoc to write a class documentation above the class definition. This documentation will be visible in the service panel and the library documentation in future versions.
Java Methods
Use Javadoc to write a method documentation above the method definition.
Use the
@param
syntax to describe parameters, e.g. what information is required or returned.Use
@return
to describe return values.Name and order of parameters in the Javadoc must match the name and order of parameters in the Java implementation.
In the Designer, you can find this piece of documentation on the library documentation page and in the service panel when hovering over the operation:
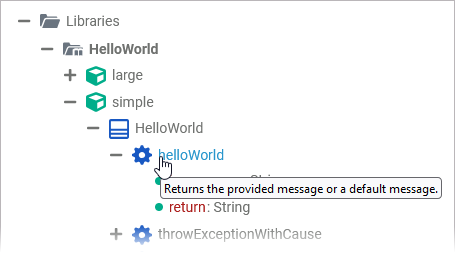
Currently, this is supported for methods/library operations only. Future versions will also support parameter documentation.