Library Development
Developers can create and use their own libraries in the Designer. The main objectives when developing your own libraries are:
Splitting your service implementation into smaller chunks that can be worked on independently.
Reusing the code in multiple services.
Sharing the code with other team members.
In order to reach these goals, we highly recommend that you define some basic rules for library development before you start. Libraries are most helpful when they are easy to use. Their structure should be consistent and their content well documented. In this article, we have compiled a number of recommendations that you should consider when developing your own libraries for use in Scheer PAS Designer.
Refer to chapter Developing and Using Libraries in the Designer Guide for more information about creation and usage of libraries in the Designer.
Developing a Library
If you want to start developing a library, create a new service in your Scheer PAS Designer.
Always create a separate service for each library!
You have two options to define what contents to share with your library:
Share library content via the Attributes panel > attribute Include in Library
Share library content with the Publish Asset wizard
Refer to Creating a Library in the Designer Guide for detailed explanations on both options.
Library Structure
Make sure you structure your libraries consistently. If your library structure always follows the same rules, user can easily find their way around the library contents and developers can maintain the library more easily. We recommend using the following basic structure:
Service Panel Element | Description | Example | ||||
---|---|---|---|---|---|---|
Implementation | Fixed package to store the library implementation. | |||||
a root package | A necessary root package.
| Library | ||||
library class(es) | One or more library classes that expose the functionality of the library. | DocxGenerator | ||||
property(ies) | Properties | |||||
operation(s) | All library operations should be non-static and rely on properties of the related class. |
| ||||
If convenient, you can provide static parameterized operations. That should not be the standard case but only in addition to the non-static operations mentioned above. | ||||||
private class(es) | One or more classes that contain implementations used in the library only. If you do not want to expose some operations related to the library class, create a class <name of library class>Private to store those. |
| ||||
property(ies) | ||||||
operation(s) | ||||||
and / or | ||||||
package(s) | If necessary to group similar implementations, you can add an intermediate package level. | |||||
library class(es) | ||||||
property(ies) | ||||||
operation(s) |
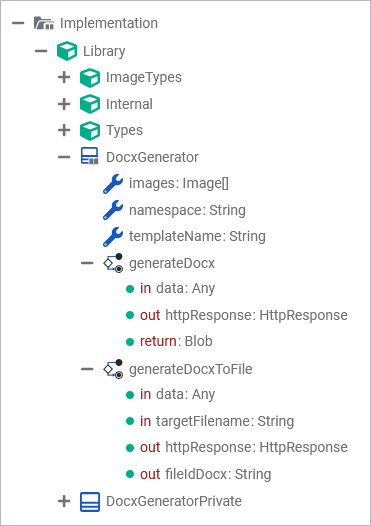
Example: PAS Library GenerateDocxPdf
Naming Conventions
For reasons of consistency, you should also define rules for naming library content. We recommend the following naming conventions (see also example above):
Element | Upper Case | Lower Case | Other Conventions | Example | |||
---|---|---|---|---|---|---|---|
package |
| Library | |||||
class | DocxGenerator | ||||||
property | templateName | ||||||
operation |
| generateDocx | |||||
| parameter | docxFile |
Interface Design
The structure of the library interface should always be built from the user perspective. When defining the interface of the library, a library developer should ask themself: What makes it easier for the user to use the library? How can I support the library user?
If you are wrapping access to a service, you should not simply expose the interface of the service.
Best Practice Examples
If some parameters are changed upon each call: Create a dedicated parameter for that (e.g. filename).
If some parameters do not need to be changed every time the library is called, and represent more of a config: Create a configuration class for this (e.g. images, namespace and templateName).
If both of the upper can apply to the parameter: Put it in both, and write a routine that populates the parameter from the configuration if not provided.
Namespace
Make sure that the library package and its sub-packages have set a namespace to distinct the contained classes from classes in the usage service that may have the same name. To do this, add the stereotype XUML Package to the packages in your library (refer to Attributes Panel > Adding More Attributes via a Stereotype in the Designer Guide):
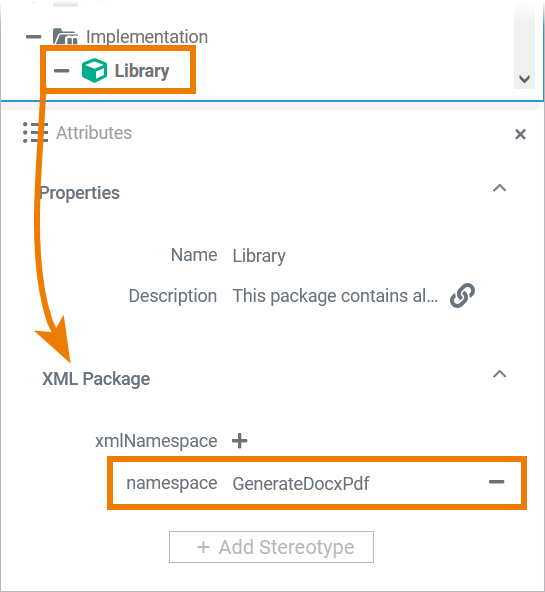
How the library structure is presented in the usage service depends on what names you assign to the namespaces.
General recommendations (see example above):
The namespace of the top-level package should be the same as the library name, e.g. GenerateDocxPdf.
The namespace of a sub-package should be the name of the top-level package followed by a dot and the name of the sub-package, e.g. GenerateDocxPdf.Types.
Changes to the Library Interface
In general, the owner of a library should, if possible, avoid changing a library in a way that breaks the interface.
For cases in which a breaking change cannot be avoided, you must define a good way of dealing with breaking changes. We recommend the following:
Deprecate instead of delete: Do not delete an outdated operation but deprecate it, e.g. in the library documentation.
Announce deletion: If you want to delete an outdated operation at some point, announce the end-of-life of this operation before deleting it in an upcoming version. This gives the library users time to prepare.
Create new operation instead of adding new parameters: Instead of introducing new parameters to existing operations, create a new operation for that.
Add migration notes: Give library users migration hints in the release notes of your library.
Library Documentation
Detailed documentation is important so that other team members can use your library quickly and easily. The Designer offers different ways to provide library documentation:
You can add documentation in the Attributes Panel.
You can add documentation in the Publish Asset Wizard.
Refer to Documenting Assets in the Designer Guide for detailed explanations on both options.
The documentation is available in several ways:
You can open the complete documentation of a library in a new tab. This is possible via the asset drawer or via the context menu of the library itself.
If you hover over library content for which documentation has been saved, this is displayed as a tooltip:
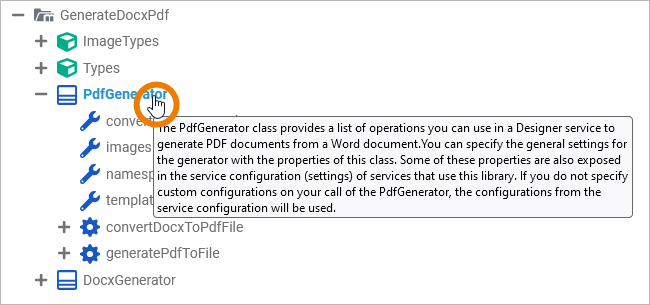
Refer to Accessing the Library’s Documentation in the Designer Guide for details.
If your libraries are well documented, users are directly supported when using the libraries in their projects. You should therefore also define your own standards for the content of the library documentation before starting library development. Here are our recommendations:
In general, when writing library documentation:
Don’t be too technical. The documentation should be easy to understand.
Write as little as possible, but as much as necessary (Remember: The content is also displayed as a tooltip!).
If you want to share the library via the asset drawer, we recommend the following:
Add a summary that briefly explains the intended use cases of the library.
In the library description (that can contain more information about the intended use cases and the usage of the library), provide release notes. Whenever you update a library, the release notes should contain information about the changes made.
Regarding the documentation of library content, our recommendations are:
Library Element | Documentation Recommendations | Example from PAS Library GenerateDocxPdf |
---|---|---|
Class | Add information about the elements that the class provides and - if necessary - about configuration details. | The PdfGenerator class provides a list of operations you can use in a Designer service to generate PDF documents from a Word document. You can specify the general settings for the generator with the properties of this class. Some of these properties are also exposed in the service configuration (settings) of services that use this library. If you do not specify custom configurations on your call of the PdfGenerator, the configurations from the service configuration will be used. |
Operation | Explain what the operation does. | Convert a given Word document to a PDF file. The generated file is stored to the File Storage. The id of the file in the file storage is returned. |
Parameter |
Parameters are displayed in a lucid table on the documentation page, containing name, type and description. | Input: Output: |
Property | Specify the expected data. Properties are displayed in a lucid table on the documentation page, containing name, type and description. | Provide a list of images that should be set into placeholders in the template file. See class Image for more details on how to provide the images. |
Error Handling
A library should pass on errors to the service that uses the library. This generally happens automatically. In the case of adapter calls, the adapter's responses are not passed on automatically. In these cases, you must catch the error in the library, read the response (or at least make the attempt to read it) and then throw the error again so that it is passed on to the service.
REST Adapter Calls
Libraries should handle REST adapter calls as follows:
Catch the REST adapter call.
Try to parse the response body.
Re-throw the exception with properties of the error object from the response body:
error domain | <name of the library> | DocxGenerator |
error code | HTTP status code | 500 |
description | HTTP status message | Errors in template |
Related Documentation: