Calling xUML Operations from JavaScript
From within your JavaScript implementation of a Designer operation, you can call other Designer operations. Assume in your Designer service model you have a the following class Product having two operations updateStock and getStock (static):

Having an object aProduct of type Product, you can access the operation in your JavaScript operation like
aProduct.updateStock(aQuantity);
You can call static class operations like getStock directly on the class itself:
Product.getStock("0024", aQuantity);
Use the class name (Product) to identify the operation in this case.
Particularities
Regarding the implementation of xUML operation calls in your JavaScript, you must respect the particularities that are described in this section.
Input Parameters
The types of provided input parameters must exactly match the type the operation requires.
There is no type conversion done.
Related to the example above, this means
Good Cases
CODEaProduct.updateStock(560.0); aProduct.updateStock(560); aProduct.updateStock(aQuantity); // aQuantity being a number Product.updateStorageSystem("00024", 1300.5); Product.updateStorageSystem(aProduct, aQuantity); // aProduct being a string, and aQuantity being a number
Bad Cases
CODEaProduct.updateStock("560.0"); let aQuantity = "560"; aProduct.updateStock(aQuantity); Product.updateStorageSystem(24, 1300.0);
Output Parameters
JavaScript does not have the concept of “output” parameters, but xUML operations have. In order to provide this functionality with JavaScript operations, all output parameters are consolidated into one JavaScript object. The following is valid for these objects:
This output object must be provided by the user if the operation has output parameters.
It must be added as the last parameter to the call.
The name of the output object is not relevant.
Output parameters will be added as properties to the output object.
In case the output object is not empty on a call:
Object properties matching a parameter name will be overwritten.
An additional property will be created if there is no property matching a parameter name.
Other properties will stay untouched.
We recommend using an empty output object.
Having an object aProduct of type Product, you can access an operation with output parameters (getStock) like:
const outputObject = {};
aProduct.getStock("0024", outputObject);
At his point, the output parameter quantity is available via the outputObject:
let aQuantity = outputObject.quantity;
The JavaScript feature called “destructuring assignment” is perfect for extracting multiple output values from the output object.
Return Parameter
The return parameter, in this context, behaves as expected:
let aQuantity = aProduct.getStock("0024");
Operation Overload Resolution
xUML operations know the concept of overloading operations. You can have the same operation multiple times, implemented with different signatures (different sets of parameters). Using such an operation in your JavaScript implementation, the xUML Runtime will try to match the call to known operation signatures. This implies the following:
The matching relies only on input parameters (and the presence of the output parameter) given in the right order. For a successful match:
The number of parameters must be the same as declared.
Their types must match.
The output object must be given (as the last parameter) if any output parameters are declared in the model.
The return parameter does not influence the overload resolution.
If no matching signature or multiple matching signatures are found, a TypeError is thrown during the execution of the JavaScript.
Operation Binding
Static operations are bound according to the static type of the target object (static binding, refer to Customizing Classes for more). Virtual operations are bound according to the dynamic type of the target object (dynamic binding, see Customizing Classes > Interface Example).
The ACME example company has implemented the following Product_Mapping class:
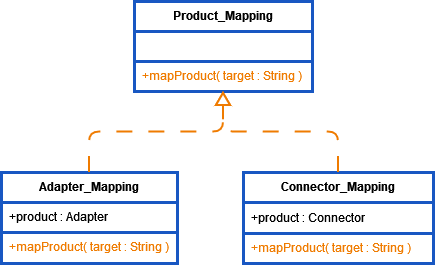
Operation mapProduct( target : String ) is implemented by two classes: Adapter_Mapping and Connector_Mapping. Depending on which of the two types is provided, the xUML Runtime uses a different implementation of mapProduct.
This means to an object declared as Product_Mapping, but currently holding a Connector_Mapping, applies the following:
aConnector_Mapping.mapProduct(target)
calls the virtual operation specified on the Connector_Mapping class.
This does not apply to static operations. Static operations are always called on the class given with the call (static type), not the derived class (dynamic type).
Exceptions
Exceptions thrown in the xUML operations will be passed to the calling JavaScript operation. In the JavaScript operation, they manifest themselves as JavaScript exceptions that you can handle with try...catch
blocks.
Unhandled exceptions will terminate the execution of the JavaScript and be passed to the Designer model to be handled there.
Current Limitations
Currently, to be able to call operations:
the static type of the target object must not be an Interface or Abstract class
the operation cannot be abstract within the static type of the target object